图书介绍
C语言参考手册 第5版 英文版【2025|PDF|Epub|mobi|kindle电子书版本百度云盘下载】
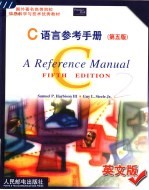
- (美)Samuel P.Harbison,Guy L.Steele Jr. 著
- 出版社: 北京:人民邮电出版社
- ISBN:7115111944
- 出版时间:2003
- 标注页数:533页
- 文件大小:16MB
- 文件页数:551页
- 主题词:C语言-程序设计-高等学校-教材-英文
PDF下载
下载说明
C语言参考手册 第5版 英文版PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
Contents1
PART 1 The C Language1
1 Introduction3
1.1 The Evolution of C3
1.2 Which Dialect of C Should You Use?6
1.3 An Overview of C Programming7
1.4 Conformance8
1.5 Syntax Notation9
2 Lexical Elements11
2.1 Character Set11
2.2 Comments18
2.3 Tokens20
2.4 Operators and Separators 20
2.5 Identifiers21
2.6 Keywords23
2.7 Constants24
2.8 C++ Compatibility38
2.9 On Character Sets,Repertoires,and Encodings39
2.10 Exercises41
3 The C Preprocessor43
3.1 Preprocessor Commands43
3.2 Preprocessor Lexical Conventions44
3.3 Definition and Replacement46
3.4 File Inclusion59
3.5 Conditional Compilation61
3.6 Explicit Line Numbering66
3.7 Pragma Directive67
3.8 Error Directive69
3.9 C++ Compatibility70
3.10 Exercises71
4 Declarations73
4.1 Organization of Declarations74
4.2 Terminology75
4.3 Storage Class and Function Specifiers83
4.4 Type Specifiers and Qualifiers86
4.5 Declarators95
4.6 Initializers103
4.7 Implicit Declarations113
4.8 External Names113
4.9 C++ Compatibility116
4.10 Exercises119
5 Types123
5.1 Integer Types124
5.2 Floating-Point Types132
5.3 Pointer Types136
5.4 Array Types140
5.5 Enumerated Types145
5.6 Structure Types148
5.7 Union Types160
5.8 Function Types165
5.9 The Void Type168
5.10 Typedef Names168
5.11 Type Compatibility172
5.12 Type Names and Abstract Declarators176
5.13 C++ Compatibility178
5.14 Exercises179
6 Conversions and Representations181
6.1 Representations181
6.2 Conversions188
6.3 The Usual Conversions194
6.4 C++ Compatibility200
6.5 Exercises201
7 Expressions203
7.1 Objects,Lvalues,and Designators203
7.2 Expressions and Precedence204
7.3 Primary Expressions207
7.4 Postfix Expressions210
7.5 Unary Expressions219
7.6 Binary Operator Expressions227
7.7 Logical Operator Expressions243
7.8 Conditional Expressions244
7.9 Assignment Expressions246
7.10 Sequential Expressions249
7.11 Constant Expressions250
7.12 Order of Evaluation253
7.13 Discarded Values255
7.14 Optimization of Memory Accesses256
7.15 C++ Compatibility257
7.16 Exercises258
8 Statements259
8.1 General Syntactic Rules for Statements260
8.2 Expression Statements260
8.3 Labeled Statements261
8.4 Compound Statements262
8.5 Conditional Statements264
8.6 Iterative Statements266
8.7 Switch Statements274
8.8 Break and Continue Statements277
8.9 Return Statements279
8.10 Goto Statements280
8.11 Null Statements281
8.13 Exercises282
8.12 C++ Compatibility282
9 Functions285
9.1 Function Definitions286
9.2 Function Prototypes289
9.3 Formal Parameter Declarations295
9.4 Adjustments to Parameter Types298
9.5 Parameter-Passing Conventions299
9.6 Agreement of Parameters300
9.7 Function Return Types301
9.8 Agreement of Return Types302
9.9 The Main Program303
9.10 Inline Functions304
9.11 C++ Compatibility306
9.12 Exercises307
PART 2 The C Libraries309
10 Introduction to the Libraries311
10.1 Standard C Facilities312
10.2 C++ Compatibility313
10.3 Library Headers and Names316
11 Standard Language Additions325
11.1 NULL,ptrdiff_t,size_t,offsetof325
11.2 EDOM,ERANGE,EILSEQ,errno,strerror,perror327
11.3 bool,false,true329
11.4 va_list,va_start,va_arg,va_end329
11.5 Standard C Operator Macros333
12 Character Processing335
12.1 isalnum,isalpha,iscntrl,iswalnum,iswalpha,iswcntrl336
12.2 iscsym,iscsymf338
12.3 isdigit,isodigit,isxdigit,iswdigit,iswxdigit338
12.4 isgraph,isprint,ispunct,iswgraph,iswprint,iswpunct339
12.5 islower,isupper,iswlower,iswupper340
12.6 isblank,isspace,iswhite,iswspace341
12.7 toascii341
12.8 toint342
12.9 tolower,toupper,towlower,towupper342
12.10 wctype_t,wctype,iswctype343
12.11 wctrans_t,wctrans344
13 String Processing347
13.1 strcat,stmcat,wcscat,wcsncat348
13.2 strcmp,strncmp,wcscmp,wcsncmp349
13.3 strcpy,strncpy,wcscpy,wcsncpy350
13.4 strlen,wcslen351
13.5 strchr,strrchr,wcschr,wcsrchr351
13.6 strspn,strcspn,strpbrk,strrpbrk,wcsspn,wcscspn,wcspbrk352
13.7 strstr,strtok,wcsstr,wcstok354
13.8 strtod,strtof,strtold,strtol,strtoll,strtoul,strtoull355
13.9 atof,atoi,atol,atoll356
13.10 strcoll,strxfrm,wcscoll,wcsxfrm356
14 Memory Functions359
14.1 memchr,wmemchr359
14.2 memcmp,wmemcmp360
14.3 memcpy,memccpy,memmove,wmemcpy,wmemmove361
14.4 memset,wmemset362
15 Input/Output Facilities363
15.1 FILE,EOF,wchar_t,wint_t,WEOF365
15.2 fopen,fclose,fflush,freopen,fwide366
15.3 setbuf,setvbuf370
15.4 stdin,stdout,stderr371
15.5 fseek,ftell,rewind,fgetpos,fsetpos372
15.6 fgetc,fgetwc,getc,getwc,getchar,getwchar,ungetc,ungetwc374
15.7 fgets,fgetws,gets376
15.8 fscanf,fwscanf,scanf,wscanf,sscanf,swscanf377
15.9 fputc,fputwc,putc,putwc,putchar,putwchar385
15.10 fputs,fputws,puts386
15.11 fprintf,printf,sprintf,snprintf,fwprintf,wprintf,swprintf387
15.12 v[x]printf,v[x]scanf401
15.13 fread,fwrite402
15.14 feof,ferror,clearerr404
15.15 remove,rename404
15.16 tmpfile,tmpnam,mktemp405
16 General Utilities407
16.1 malloc,calloc,mlalloc,clalloc,free,cfree407
16.2 rand,srand,RAND_MAX410
16.3 atof,atoi,atol,atoll 1411
16.4 strtod,strtof,strtold,strtol,strtoll,strtoul,strtoull412
16.5 abort,atexit,exit,_Exit,EXIT_FAILURE,EXIT_SUCCESS414
16.6 getenv415
16.7 system416
16.8 bsearch,qsort417
16.9 abs,labs,llabs,div,ldiv,lldiv419
16.10 mblen,mbtowc,wctomb420
16.11 mbstowcs,wcstombs422
17 Mathematical Functions425
17.1 abs,labs,llabs,div,ldiv,lldiv426
17.2 fabs426
17.3 ceil,floor,lrint,llrint,lround,llround,nearbyint,round,rint,trunc427
17.4 fmod,remainder,remquo428
17.5 frexp,ldexp,modf,scalbn429
17.6 exp,exp2,expml,ilogb,log,log10,loglp,log2,logb430
17.7 cbrt,fma,hypot,pow,sqrt432
17.8 rand,srand,RAND_MAX432
17.9 cos,sin,tan,cosh,sinh,tanh433
17.10 acos,asin,atan,atan2,acosh,asinh,atanh434
17.12 Type-Generic Macros435
17.11 fdim,fmax,fmin435
17.13 erf,erfc,lgamma,tgamma439
17.14 fpclassify,isfinite,isinf,isnan,isnormal,signbit440
17.15 copysign,nan,nextafter,nexttoward441
17.16 isgreater,isgreaterequal,isless,islessequal,islessgreater,isunordered442
18 Time and Date Functions443
18.1 clock,clock_t,CLOCKS PER_SEC,times443
18.2 time,time_t445
18.3 asctime,ctime445
18.4 gmtime,localtime,mktime446
18.5 difftime447
18.6 strftime,wcsftime448
19 Control Functions453
19.1 assert,NDEBUG453
19.4 setjmp,longjmp,jmp_buf454
19.2 system,exec454
19.3 exit,abort454
19.5 atexit456
19.6 signal,raise,gsignal,ssignal,psignal456
19.7 sleep,alarm458
20 Locale461
20.1 setlocale461
20.2 localeconv463
21 Extended Integer Types467
21.1 General Rules467
21.2 Exact-Size Integer Types470
21.3 Least-Size Types of a Minimum Width471
21.4 Fast Types of a Minimum Width472
21.5 Pointer-Size and Maximum-Size Integer Types473
21.7 imaxabs,imaxdiv,imaxdiv_t474
21.6 Ranges of ptrdiff_t,size_t,wchar_t,wint_t,and sig_atomic_t474
21.8 strtoimax,strtouimax475
21.9 wcstoimax,wcstoumax475
22 Floating-Point Environment477
22.1 Overview477
22.2 Floating-Point Environment478
22.3 Floating-Point Exceptions479
22.4 Floating-Point Rounding Modes481
22.5 Floating-Point Expression Contraction481
23 Complex Arithmetic483
23.1 Complex Library Conventions483
23.2 complex,_Complex_I,imaginary,_Imaginary_I,I484
23.3 CX_LIMITED_RANGE484
23.4 cacos,casin,catan,ccos,csin,ctan485
23.5 cacosh,casinh,catanh,ccosh,csinh,ctanh486
23.6 cexp,clog,cabs,cpow,csqrt487
23.7 carg,cimag,creal,conj,cproj488
24 Wide and Multibyte Facilities489
24.1 Basic Types and Macros489
24.2 Conversions Between Wide and Multibyte Characters490
24.3 Conversions Between Wide and Multibyte Strings491
24.4 Conversions to Arithmetic Types493
24.5 Input and Output Functions493
24.6 String Functions493
24.7 Date and Time Conversions494
24.8 Wide-Character Classification and Mapping Functions494
A The ASCII Character Set497
B Syntax499
C Answers to the Exercises513
Index521
热门推荐
- 64037.html
- 2660420.html
- 2407030.html
- 297797.html
- 326309.html
- 152092.html
- 1473465.html
- 858416.html
- 1703385.html
- 994457.html
- http://www.ickdjs.cc/book_3512885.html
- http://www.ickdjs.cc/book_2671591.html
- http://www.ickdjs.cc/book_1159582.html
- http://www.ickdjs.cc/book_1606799.html
- http://www.ickdjs.cc/book_2692538.html
- http://www.ickdjs.cc/book_2878008.html
- http://www.ickdjs.cc/book_3139783.html
- http://www.ickdjs.cc/book_779836.html
- http://www.ickdjs.cc/book_2473677.html
- http://www.ickdjs.cc/book_2501796.html